Assumptions you shouldn't make as a developer
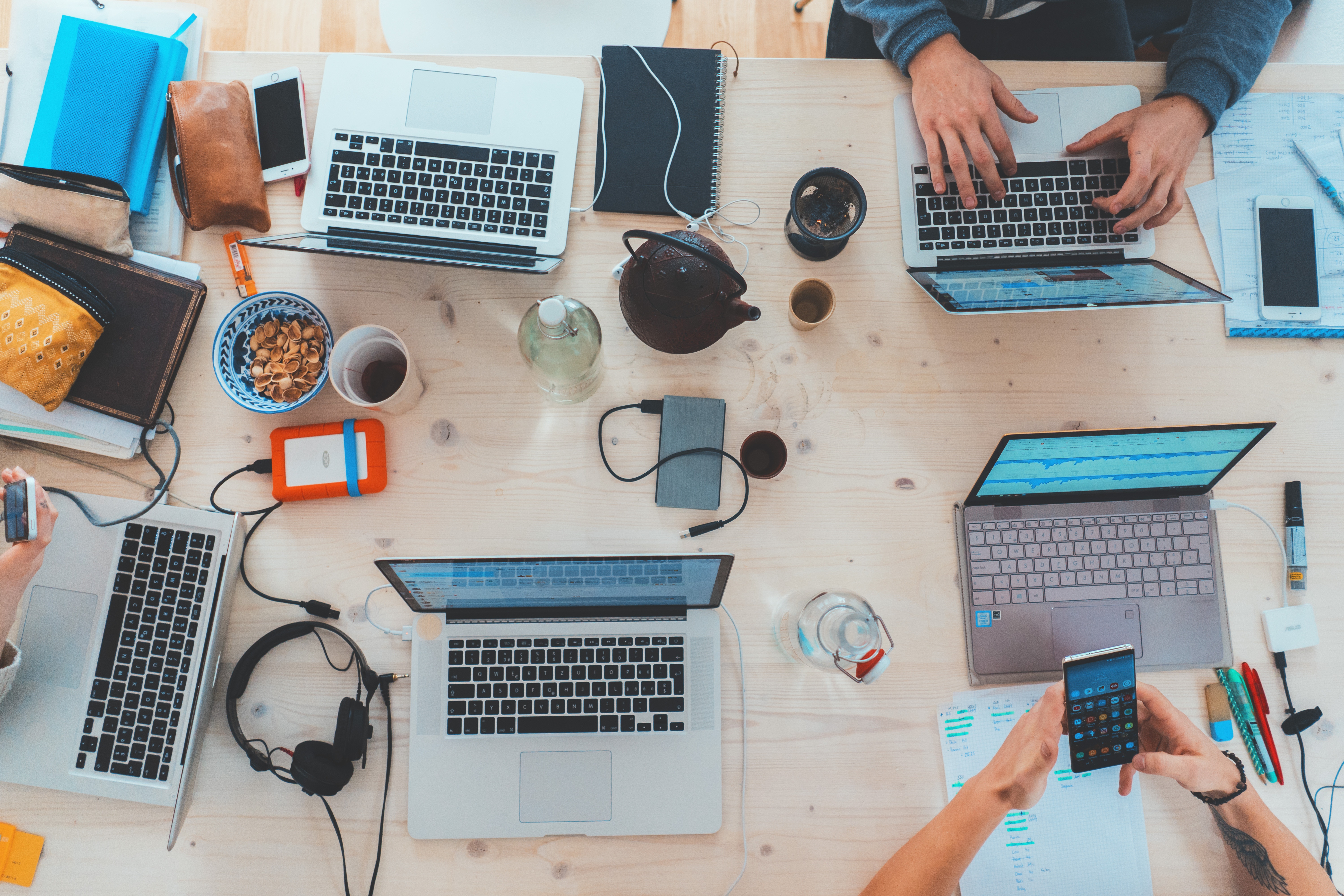
I have worked as a software engineer for almost 4 years now and it still excites me everyday in the morning that I get to work on projects that make impacts to our customers at Article. I have learned a lot of new skills and one of the things I have learned is that making assumptions can sometimes have negative impacts on the project. The following advices are not just for developers but may also be valid for other people.
Your code will work
If you are calling an API endpoint, you can most certainly assume the data will be in this certain format since that how it was designed. However, we cannot assume the data will always be there so we need to check for errors. If you are using async / await with fetch:
try {
await fetch(/* url */).then(res => res.json());
} catch (error) {
// handle the error
}
Even if we could assume the format, we can’t assume that each property in that JSON object has the data we have. For example you are calling a users endpoint that returns a list of users which contains user object that may have first name, last name, and age. If you start looping it and manipulating the data without checking if data is there, your app will crash.
let names = users.map(user => {
return user.firstName.toLowerCase() + user.lastName.toLowerCase();
});
The above code will fail if firstName or lastName are not available as strings. You can never be too careful. If the app crashes because of an error we forgot to check, this user may never return to use your product and you lose a user.
People have received your message
You may need to require something from your colleagues. They can be the designer for UI changes or icons, or copy writer for text, or experiment results from Data Team. Don't assume they have received your message. You need to follow up to make sure the message has been delivered. Otherwise you may wait forever for something that is not going to happen and eventually the project may be delayed and you may be held responsible for it. Don't feel like you are rushing other people or bothering other people. Instead, you are reminding other people and with their help you can finish your project and everybody can benefit from it.
They have forgot about it
Contrary to the prior point, if your manager asks you to do something but never follow up, don't assume your manager has forgot about it. They trust you and think you are accountable so they assign you a task. Now it becomes your responsibility to conclude the task with a solution or close the ticket with your answer. Don't leave it hanging and assuming nobody remembers this. You may soon lose people's trust in you. If you couldn't do it in the first place then let them know immediately, discuss the urgency of the task, and maybe assign other people to do it if applicable or wait till you finish your tasks.
People understand what you mean
When there are new engineers or when new people joining your project, you might need to explain things to them. Please do make sure they understand what you are saying. Don't assume they just understand every single word you say and move on with your work. Don't be the person that thinks they are here to take your jobs so you intentionally sabotage them. You bring enormous value to the team if you can get the new people on board quickly, help them contribute to the team quickly, and get them up to speed quickly. Your manager will appreciate you. Write the documents if you need to so the information doesn't stay in your head but in the company's knowledge base.